Python script (segmentation)
Segmentation of measurement using an external python script.
On a Windows machine, you need to install Python version 3 or higher and the py
Python launcher
First, select Segmentation as node type and then select Python script as the method. Select the python script file using the Browse button or type the path to the script file directly.
Parameters
Python file
Select python file.
Python interpreter (optional)
Select a custom interpreter executable, empty will use py
on Windows and python
command on Linux and macOS.
Min area
The minimum number of pixels for an object to be included.
Max area
The maximum number of pixels for an object to be included.
If 0 no maximum area is defined.
Object filter
Use an expression to further exclude unwanted objects based on shape.
Properties that can be used for the Expression:
Area
Length
Width
Circumference
Regularity
Roundness
Angle
D1
D2
X
Y
MaxBorderDistance
BoundingBoxArea
For details on each available property see: Object properties Details
Shrink
Takes away x numbers of pixels at the borders of the objects included in images.
Separate
Normal
Can have both separated and combined objects.
Separate adjacent objects
All objects are defined separately.
Merge all objects into one
All objects are defined as one.
Merge all objects per row
All objects per row segmentation are defined as one.
Merge all objects per column
All objects per column segmentation are defined as one.
Link
Only visible when applicable
Link output objects from two or more segmentations to top segmentation. Descriptors can then be added to the common object output and will be calculated for objects from all segmentations.
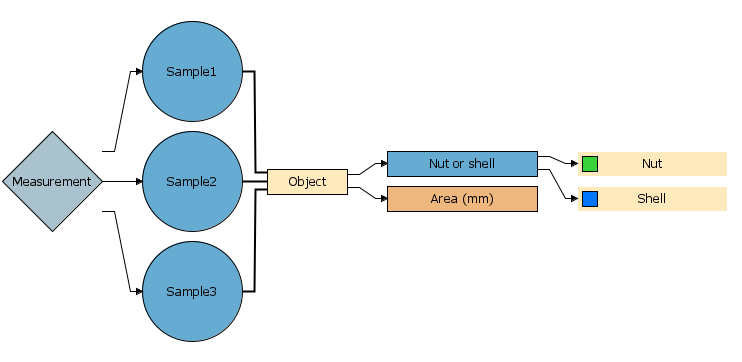
Descriptors after object will be calculated for all three segmentations (Sample1, Sample2 and Sample3)
The segmentations must be at same level to be available for linking.
Simple python script for segmentation
import sys
try:
with open(sys.argv[1], "r") as f:
frames = f.read().splitlines()
result = []
for frame in frames:
pixels = [float(x) for x in frame.split(";")]
if pixels and pixels[1] < 0.5:
result.append(1)
else:
result.append(0)
print(result)
except Exception as e:
_, _, exc_tb = sys.exc_info()
print(f"Error: {e}, at line {exc_tb.tb_lineno}")
Example input to the script
The input to the script is saved to a temp file. The path to the temp file is then passed as a parameter to the python script. The script has to read this file to collect the input from Breeze. This is since the max parameter length is too short to hold all data needed. This type of segmentation is not recommended if performance is an priority as it has a lot of overhead.
17;16;19
15;14;13
15;14;16
....
Ending with an empty row.
Example output from the script
The output has to be printed from the python script to be returned to Breeze: print(result)
.
[1, 1, 0]
Everything inside the yellow line has now been selected as a sample.